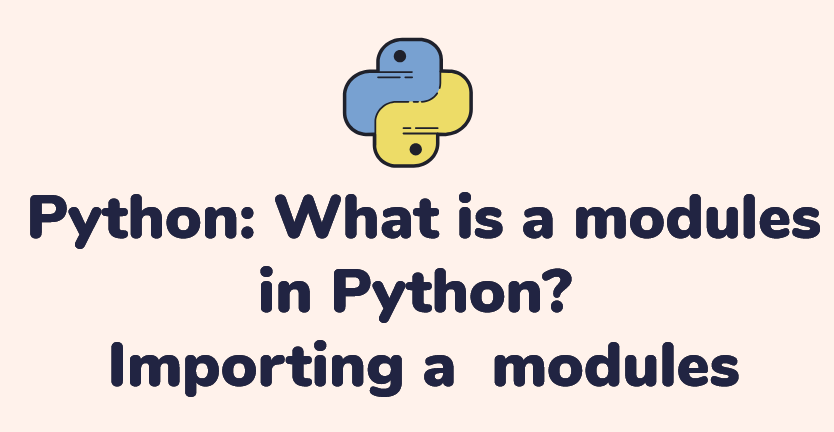
What is a modules in Python?
Contents
In this artical we are discuss about what is modules and how to use modules in Python. The propensity of computer code is to expand. We can conclude that code that remains static is most likely abandoned or utterly worthless. Real, desired, and extensively used code evolves throughout time in response to changing user expectations and demands, which follow their own rhythms.
If a code cannot adapt to the needs of users, it will be swiftly forgotten and replaced with a new, improved, and more flexible code. Never assume that any of your programs will ever be finished, and be ready for this. Following the initial bug report, the completion is a transitional state that typically goes by fast. Python serves as a nice illustration of how the rule operates.
Indeed, the challenge of expanding code is growing. More code always translates to more maintenance. Just as it is always easier to identify a mechanical breakage in simpler, smaller machinery, it is also always easier to search for flaws in smaller, simpler code.
Furthermore, you may want—or rather, you will be forced—to divide the code into multiple parts that are implemented in parallel by a few, a dozen, several dozen, or even several hundred individual developers when the code being created is expected to be extremely large (you can use the total number of source lines as a useful, if not very accurate, measure of a code’s size).
To successfully finish a software project of this nature, you must possess the flow that will enable you to:
- divide all the tasks among the developers;
- join all the created parts into one working whole.
How to use modules in Python?
Modules in Python mean that a file that containing Python definition and statements, which can be imported when it’s necessary. That is call modules also. Simple definition of modules in Python mean that part of code which we can use when it’s required, it’s call modules.
There are two different issues to handling the modules in Pythons.
when you want to use an already existing module, written by someone else, or created by yourself during your work on some complex project – in this case you are the module’s user
when you want to create a brand new module, either for your own use, or to make other programmers’ lives easier – you are the module’s supplier.
A module is first recognized by its name. You must be aware of the name of each module in order to use it. Python comes with a variety of modules, some of which are fairly huge. They can be compared to “Python extra equipment”.
Namespace in Python
Let’s continue with important term: namespace in python. A namespace is a space in which some name are exist and that name are not conflict with each other.

There are not two different object with same name. Each namespace group that consider as one social group. In above example each object has it’s own group name and object name is unique among all group. This may mean that some names may disappear when any other entity of an already known name enters the namespace. If you want to access any of the object you need to use it’s parent name and we can use alias for access the object as well.
Import a modules in Python
Python provides several ways to import modules in python, allowing you to access functions, classes, and variables defined in other files. Here are the common methods for importing modules in pythons
Import the entire module:
When you import an entire modules in python, you bring in all the functions, classes, and variables defined in that module. This is useful when you need to access multiple items from the module.
import module_name
Suppose you have a module named math_operations.py
.
# math_operations.py
def add(a, b):
return a + b
def subtract(a, b):
return a - b
pi = 3.14159
You can import the entire module and access its contents like this
import math_operations
result = math_operations.add(5, 3)
print(result) # Output: 8
print(math_operations.pi) # Output: 3.14159
Above you can see that if you want to use any of the function of math_operation.py you should use function namespace first.
Import Specific Functions or Variables
If you only need specific items from a module, you can import them directly. This method helps to avoid namespace clutter and makes the code cleaner.
from module_name import function_name, variable_name
In this example, you can use add
and pi
directly without prefixing them with the module name.
from math_operations import add, pi
result = add(5, 3)
print(result) # Output: 8
print(pi) # Output: 3.14159
Import a Module and Assign It an Alias
To make your code more readable or to avoid name conflicts, you can import a module and assign it an alias. This is especially useful for modules with long names. Aliasing causes the module to be identified under a different name than the original. This may shorten the qualified names, too.
import module_name as alias
The “module” identifies the original module’s name while the “alias” is the name you wish to use instead of the original.
Note: as
is a keyword.
import math_operations as mo
result = mo.add(5, 3)
print(result) # Output: 8
print(mo.pi) # Output: 3.14159
Here, math_operations
is aliased as mo
, making it quicker to reference in the code.
Import All Names from a Module
If you need all functions, classes, and variables from a module, you can import everything at once. However, this method is generally discouraged because it can lead to name conflicts and make the code harder to read.
from module_name import *
In this case, all public names from math_operations
are imported into the current namespace, allowing you to use them directly. Be cautious with this approach, especially in larger projects or when working with multiple modules in Python.
from math_operations import *
result = add(5, 3)
print(result) # Output: 8
print(pi) # Output: 3.14159
By understanding these different methods of importing modules in Python, you can better organize your code, enhance its readability, and reduce the chances of name conflicts. Here’s a quick recap of the import methods discussed