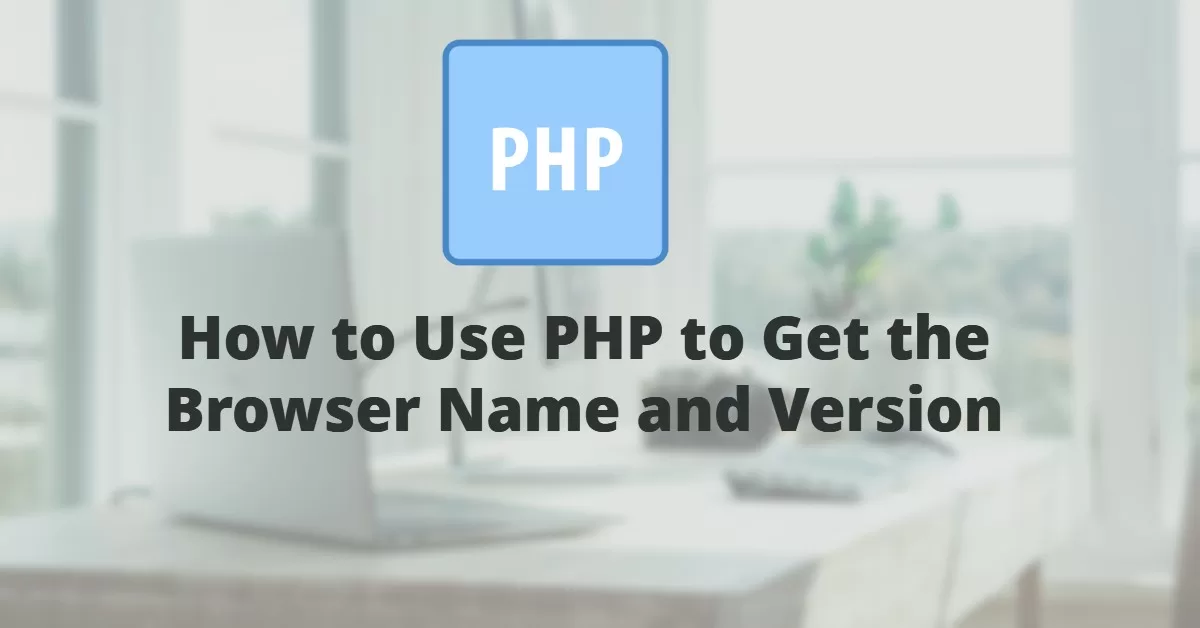
How to Use PHP to Get the Browser Name and Version
Contents
When developing a website or web application, knowing the user’s get the browser name and version can help optimize the user experience. As a server-side scripting language, PHP offers multiple methods for retrieving this data. In this post, we’ll look at how to use PHP to obtain the exact browser name and version and include it easily into your code.
Get the Browser Name and Version
The $_SERVER['HTTP_USER_AGENT']
variable in PHP contains the user-agent string sent by the client’s browser. This string includes details about the browser, operating system, and device. Parsing this string allows you to extract the browser name and version.
Using PHP to Parse the User-Agent String
Here’s a step-by-step guide to retrieve the browser name and version using PHP:
<?php
function getBrowserInfo() {
$userAgent = $_SERVER['HTTP_USER_AGENT'];
$browserInfo = "Unknown Browser";
$browsers = [
'Edge' => 'Edge',
'Chrome' => 'Chrome',
'Safari' => 'Safari',
'Firefox' => 'Firefox',
'Opera' => '(Opera|OPR)',
'Internet Explorer' => 'MSIE|Trident'
];
foreach ($browsers as $browser => $pattern) {
if (preg_match("/{$pattern}/i", $userAgent, $matches)) {
$version = "Unknown Version";
if (preg_match("/{$pattern}[\/\s]?([0-9.]+)/i", $userAgent, $versionMatches)) {
$version = $versionMatches[1];
}
$browserInfo = $browser . ' ' . $version;
break;
}
}
return $browserInfo;
}
// Example usage
echo "Your browser is: " . getBrowserInfo();
?>
Explanation
$_SERVER['HTTP_USER_AGENT']
: This PHP superglobal retrieves the user-agent string from the client’s request headers.- Browser Patterns: A list of common browsers and their patterns is defined for matching purposes.
- Regular Expressions: Patterns are used to find browser names and versions within the user-agent string.
- Version Matching: Another regular expression extracts the version number associated with the detected browser.
Another Method to get Get the Browser Name and Version
<?php
function getBrowser()
{
$u_agent = $_SERVER['HTTP_USER_AGENT'];
$bname = 'Unknown';
$platform = 'Unknown';
$version= "";
//First get the platform?
if (preg_match('/linux/i', $u_agent)) {
$platform = 'linux';
}
elseif (preg_match('/macintosh|mac os x/i', $u_agent)) {
$platform = 'mac';
}
elseif (preg_match('/windows|win32/i', $u_agent)) {
$platform = 'windows';
}
// Next get the name of the useragent yes seperately and for good reason
if(preg_match('/MSIE/i',$u_agent) && !preg_match('/Opera/i',$u_agent))
{
$bname = 'Internet Explorer';
$ub = "MSIE";
}
elseif(preg_match('/Firefox/i',$u_agent))
{
$bname = 'Mozilla Firefox';
$ub = "Firefox";
}
elseif(preg_match('/Chrome/i',$u_agent))
{
$bname = 'Google Chrome';
$ub = "Chrome";
}
elseif(preg_match('/Safari/i',$u_agent))
{
$bname = 'Apple Safari';
$ub = "Safari";
}
elseif(preg_match('/Opera/i',$u_agent))
{
$bname = 'Opera';
$ub = "Opera";
}
elseif(preg_match('/Netscape/i',$u_agent))
{
$bname = 'Netscape';
$ub = "Netscape";
}
// finally get the correct version number
$known = array('Version', $ub, 'other');
$pattern = '#(?<browser>' . join('|', $known) .
')[/ ]+(?<version>[0-9.|a-zA-Z.]*)#';
if (!preg_match_all($pattern, $u_agent, $matches)) {
// we have no matching number just continue
}
// see how many we have
$i = count($matches['browser']);
if ($i != 1) {
//we will have two since we are not using 'other' argument yet
//see if version is before or after the name
if (strripos($u_agent,"Version") < strripos($u_agent,$ub)){
$version= $matches['version'][0];
}
else {
$version= $matches['version'][1];
}
}
else {
$version= $matches['version'][0];
}
// check if we have a number
if ($version==null || $version=="") {$version="?";}
return array(
'userAgent' => $u_agent,
'name' => $bname,
'version' => $version,
'platform' => $platform,
'pattern' => $pattern
);
}
// now try it
$ua=getBrowser();
$yourbrowser= "Your browser: " . $ua['name'] . " " . $ua['version'] . " on " .$ua['platform'] . " reports: <br >" . $ua['userAgent'];
print_r($yourbrowser);
?>
Detecting the browser name and version in PHP can be useful for providing tailored experiences to users. By integrating this functionality into WordPress, you can enhance compatibility, analytics, and user engagement. The provided code is simple to implement and highly customizable for various use cases.